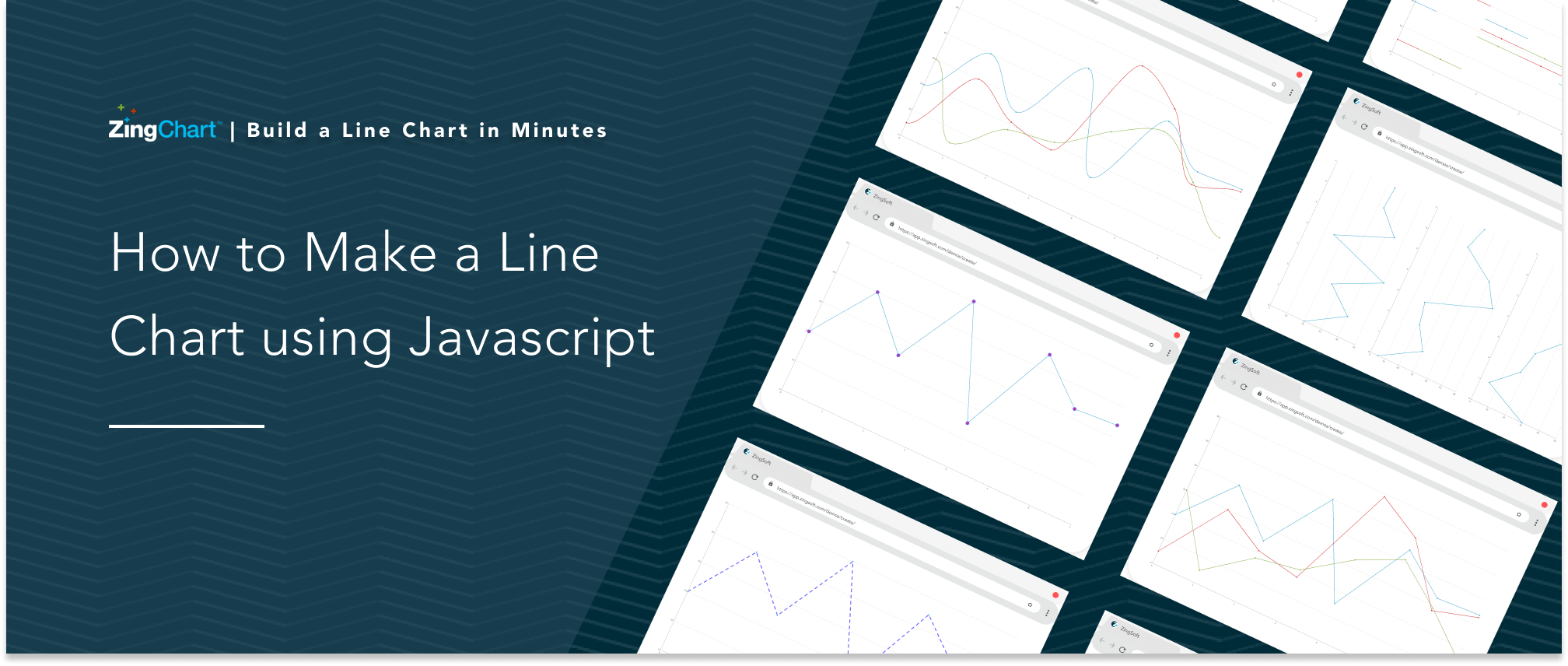
A line chart, or graph, is a highly efficient way to display data points connected by lines. Highly versatile, line charts come in three different types: simple line charts, 3-D line charts, and vertical-line charts.
This article will show you how to:
- Reference the ZingChart library
- Place your chart in your HTML page
- Add data and render your chart
- Add basic customization to your chart
Before I Begin
Before beginning my tutorial, I highly recommend signing up for ZingChart’s free Studio web-app. It’s a custom, integrated sandbox-like environment where you can test and create charts, and then share them when finished. It also provides pre-made starting templates for most of the built-in chart types, so you never need to start from scratch.
Check out the Studio and start charting!
1. Referencing the ZingChart Library
In your HTML page, reference the ZingChart library in the <head>
section of the document by adding the library <script>
call:
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>ZingChart: My Line Chart</title>
<script src="https://cdn.zingchart.com/zingchart.min.js"></script>
</head>
<body>
</body>
</html>
We’ve referenced the code from our Content Delivery Network (CDN), but you may also download the ZingChart library or use a package manager, like NPM.
2. Placing Your Chart in the Page
Before adding data to your line chart, you will need to tell ZingChart where to render the chart by referencing it within the <body>
of your code:
<!DOCTYPE html>
<html>
<head>
<meta charset=”utf-8">
<title>ZingChart: My Line Chart</title>
<script src="https://cdn.zingchart.com/zingchart.min.js"></script>
</head>
<body>
<div id="myChart"></div>
</body>
</html>
Create a <div>
container within the <body>
of your HTML page, and give it a unique id
that you will reference when rendering the chart. Simple!
3. Adding Data and Rendering Your Chart
This is where some knowledge of JavaScript is required.
In the simplest method, defining data and adding it to your chart are both done inline in a <script>
tag.
First, you assign your data and chart config to a variable, and then reference it in the render method:
<script>
var myConfig = {
type: 'line',
series: [
{ values: [20,40,25,50,15,45,33,34] },
{ values: [5,30,21,18,59,50,28,33] },
{ values: [30,5,18,21,33,41,29,15] }
]
};
zingchart.render({
id: 'myChart',
data: myConfig,
height: '100%',
width: '100%'
});
</script>
Try adding the above code within the <body>
of your HTML page. If you run this in your browser, you will have a fully functioning line chart!
Note: When adding the <script>
tag, ensure it is placed after the referenced <div id="myChart">
that renders the chart.
Check out the full line-chart demo.
So what exactly is happening above?
First, the variable myConfig
was created to store the chart’s data and configuration settings.
var myConfig = {
type: 'line',
series: [
{ values: [20,40,25,50,15,45,33,34] }, // Line 1
{ values: [5,30,21,18,59,50,28,33] }, // Line 2
{ values: [30,5,18,21,33,41,29,15] } // Line 3
]
};
The type
property is set to line and tells ZingChart which type of chart to render. ZingChart has more than 35 built-in types to choose from!
The series
property is where we define the chart’s data. It accepts an array of objects, which each represents one line in the chart. We’ve added 3 different lines to the chart, and defined its data points in the individual values
arrays.
Finally, the zingchart.render()
method builds the chart and adds the code to your referenced div
container.
zingchart.render({ // Render method to show chart on page
id: 'myChart', // Reference the id of your <div> container
data: myConfig, // Reference the 'myConfig' settings object
height: '100%', // Set the height of the chart
width: '100%' // Set the width of the chart
});
It accepts an object that has 4 properties:
id
data
height
width
height
and width
are optional if you would rather control the dimensions with CSS.
Reference the //
comments above for further information on what these do.
4. Basic Customization of Your Line Chart
Now that you have a fully-functioning line chart running in your page, there are a few easy ways to customize the look and feel of your chart. For a deeper look into the customizations available, read ZingChart’s documentation.
Changing to a Line-Chart Variant
Previously, we saw that setting the type
property to line in the configuration object rendered our data as a line chart. There are a few other values you can give type
that will render the data differently, without needing to change the data structure:
line3d
- Gives your plotted lines a 3D aspectvline
- Turns your graph vertical instead of horizontal
Setting a Plot Aspect
If you include a property called plot
, and give it a value of an object with property aspect
, you will modify how the lines themselves are rendered:
segmented
- Separates data points by connected straight linesspline
- Separates data points by connected curved linesstepped
- Separates data points by vertical and horizontal lines, resembling stepsjumped
- Separates data points by only the horizontal portion of a stepped line
var myConfig = {
type: 'line',
plot: {
aspect: 'segmented'
},
series: [
{ values: [20,40,25,50,15,45,33,34] }, // Line 1
{ values: [5,30,21,18,59,50,28,33] }, // Line 2
{ values: [30,5,18,21,33,41,29,15] } // Line 3
]
};
Changing the Styling of the Lines
To change the styling of the lines independently, add properties to the individual line-objects in the series
array:
var myConfig = {
type: 'line',
series: [
{
values: [20,40,25,50,15,45,33,34],
'line-color': #6666ff, // Hexadecimal or RGB
'line-style': 'dashed', // solid | dashed
'line-width': 5 // In pixels
},
{ values: [5,30,21,18,59,50,28,33] },
{ values: [30,5,18,21,33,41,29,15] }
]
};
Note: for property names with dashes, the key must be in quotations.
In Summary
You have learned to create and set up a line chart with your data. For more information, and to learn all that you can add to just line charts, visit the line chart page in the ZingChart documentation.
For some inspiration on charts that have already been created, visit ZingChart’s gallery, or jump directly to the line-chart examples. Feel free to hit the edit button on any of the demos, which will fork and save it to your ZingChart Studio account!
A pioneer in the world of data visualization, ZingChart is a powerful JavaScript library built with big data in mind. With over 35 chart types and easy integration with your development stack, ZingChart allows you to create interactive and responsive charts with ease.